Integrating Into a Frontend¶
The engine provides an interface to easily attach a frontend.
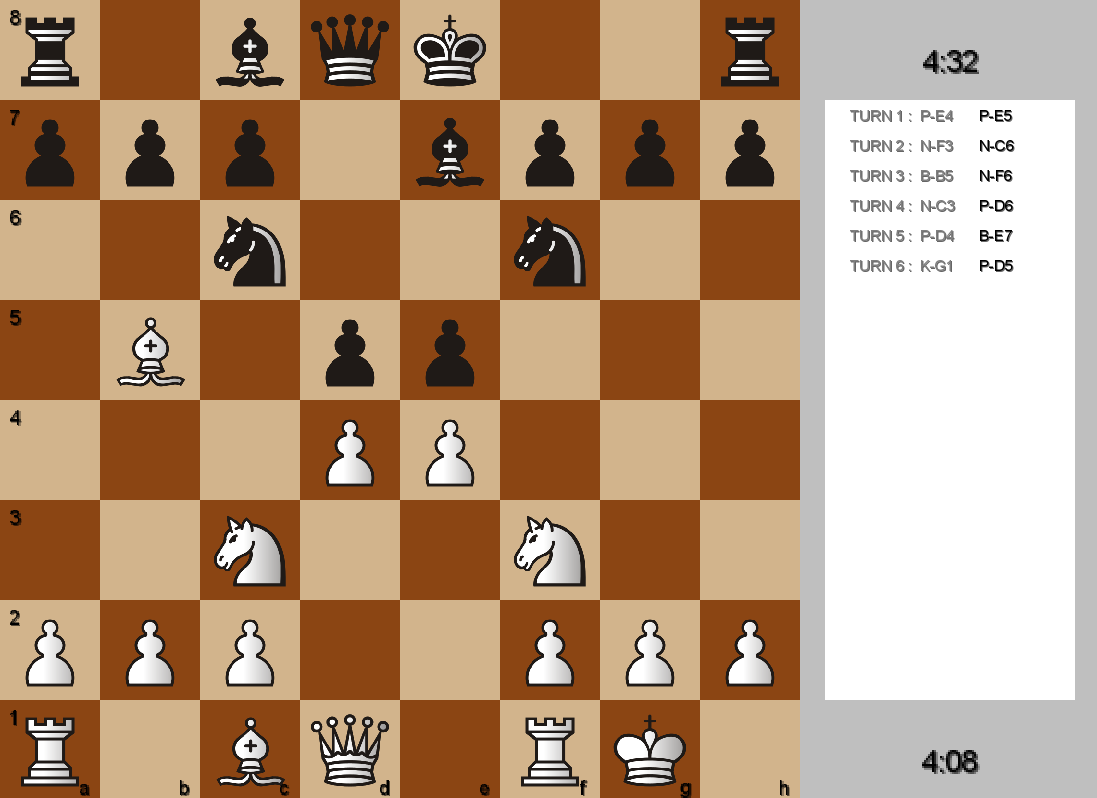
Provided example front end¶
There is an example front end already implemented as part of the engine which can be adapted to account for any extensions or changes to the library.
For our variant, we can extend this example desktop front end to play our game.
Step 1: Add images for new pieces¶
The Berlin Pawn can use the same image as a standard pawn which is already included in the library. The Alfil is usually represented as an elephant.
Navigate to the core.assets folder, and add these two images inside of it. Name them WhiteAlfil.png and BlackAlfil.png as appropriate.
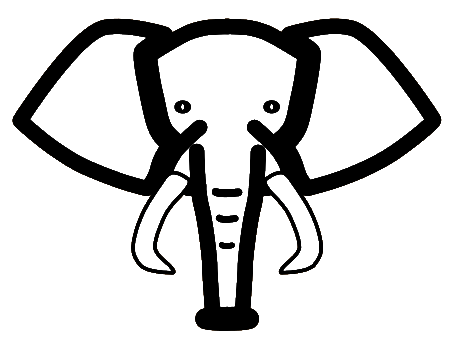
WhiteAlfil¶
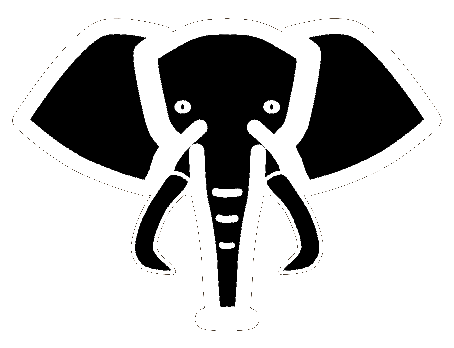
BlackAlfil¶
Step 2: Map these images to textures¶
Navigate to core.src.com.mygdx.game.assets
In TextureAssets.kt, add new enums for the BlackAlfil and WhiteAlfil with a path to the image.
enum class TextureAssets(val path: String) {
...
WhiteAlfil("WhiteAlfil.png"),
BlackAlfil("BlackAlfil.png")
}
In Textures.kt, add new variables for the WhiteAlfil and BlackAlfil textures
val blackAlfil = assets[TextureAssets.BlackAlfil]
val whiteAlfil = assets[TextureAssets.WhiteAlfil]
Step 3: Map textures to pieces¶
Navigate to core.src.com.mygdx.game.assets.Textures.kt
In the ‘whites’ mapping, add the following mappings:
Alfil::class to whiteAlfil,
BerlinWhitePawn::class to whitePawn,
In the ‘blacks’ mapping, add the following mappings:
Alfil::class to blackAlfil,
BerlinBlackPawn::class to blackPawn,
yay¶
Now when you start up the desktop applciation, you should be able to see an option for the variant you’ve created and be able to play it.